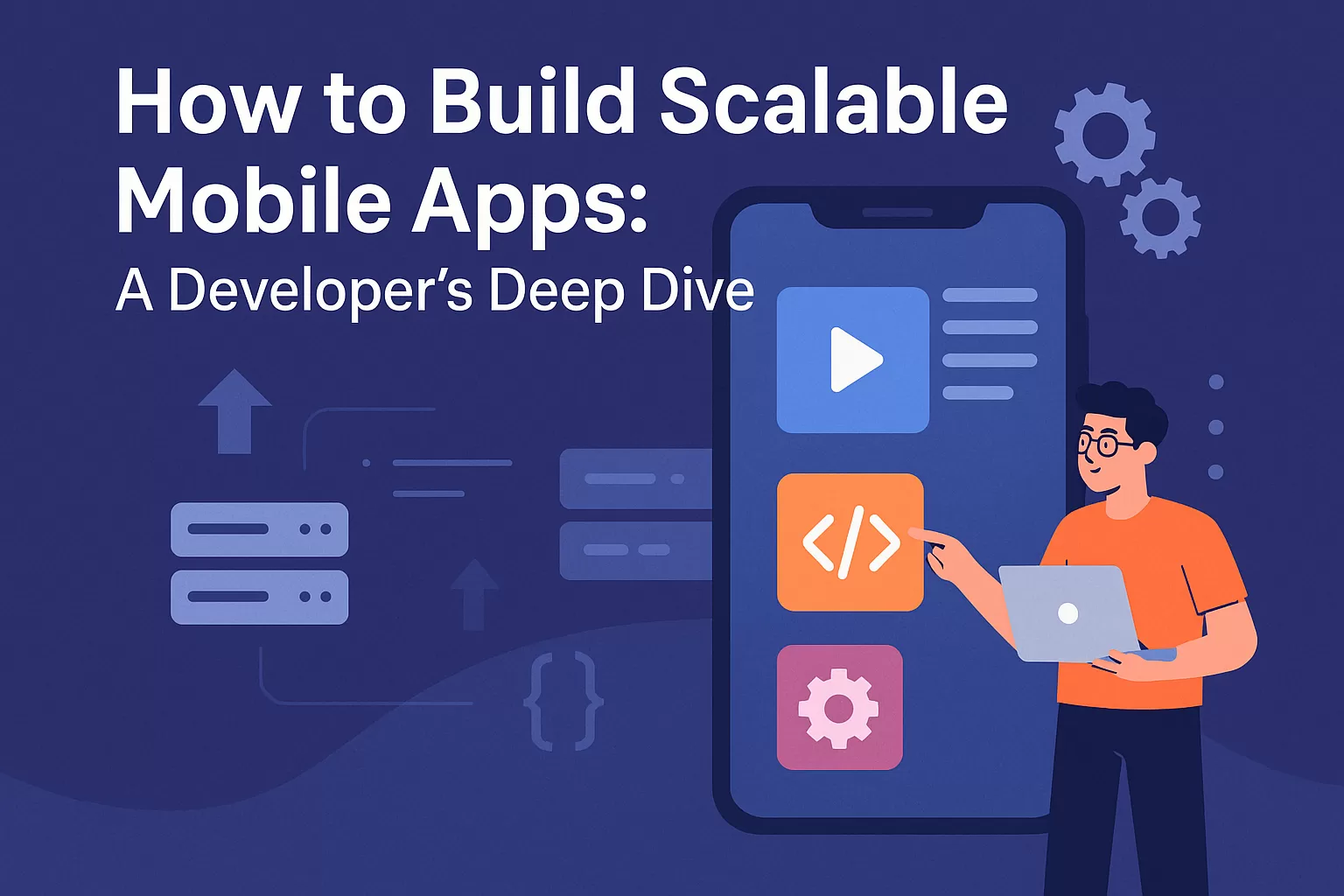
Introduction
Scalability is one of the most underestimated traits in mobile app development. Startups often focus on building MVPs quickly to validate ideas. But once your app gains traction, the pain of poor scalability begins to show — crashes, slow loading times, poor offline support, failing data sync, and poor user experience.
This article is a deep dive into how to build scalable mobile apps from day one — with the flexibility to scale when needed. It covers architectural patterns, database decisions, server-side considerations, offline-first design, real-time data handling, and more.
1. Design for Modularity, Not Monoliths
A scalable app begins with clean separation of concerns. Modular architecture ensures that your codebase doesn’t grow into an unmanageable blob. This is where design patterns like MVVM, VIPER, and Clean Architecture shine.
Real-world example: Instagram migrated from a monolithic iOS app to a modular architecture to allow faster independent deployment by teams.
Best practice: Break your app into independent modules like Auth, Feed, Messaging, Notifications. This allows teams to scale and work independently.
2. Use Scalable Mobile Architecture Patterns
When an app begins to scale, tightly coupled components can become a nightmare. Choose an architecture that promotes testability, separation, and maintainability.
Recommended Patterns:
- MVVM for iOS and Android
- Redux with Jetpack Compose or SwiftUI
- Clean Architecture (Domain, Data, UI Layers)
Code snippet (Swift — MVVM):
class UserViewModel {
@Published var user: User?
func fetchUser() {
APIManager.shared.getUser { result in
switch result {
case .success(let user): self.user = user
case .failure(let error): print(error)
}
}
}
}

3. Use Scalable Databases with Offline Support
Apps should function even in poor or no network. This means syncing data and queueing actions offline.
Options:
- Firebase Realtime Database or Firestore for real-time and scalability
- Realm or SQLite for local storage
- Couchbase Mobile for enterprise-scale offline-first apps
Case Study: WhatsApp queues all messages in a local database and syncs them via their server once internet resumes.
4. Backend Scalability Matters Too
A mobile app is only as scalable as the backend that supports it.
Key considerations:
- Use microservices or serverless (e.g., AWS Lambda, Firebase Functions)
- Implement rate limiting and caching
- Load test your API endpoints
Code snippet (Firebase Cloud Function):
exports.sendNotification = functions.firestore
.document('messages/{msgId}')
.onCreate((snap, context) => {
const message = snap.data();
// Logic to send notification
});
Real-world example: Netflix uses AWS Lambda to auto-scale backend tasks without having to manage servers manually.
5. Adopt CDN & Media Optimization for Asset Scalability
Large images, videos, and assets will slow down your app and eat up user data.
Use:
- CDNs like Cloudflare or AWS CloudFront
- Adaptive image loading libraries like Glide (Android), SDWebImage (iOS)
- Use WebP for smaller images
6. Implement Real-Time Capabilities Smartly
Scalable real-time features (chat, stock prices, live updates) can become bottlenecks if not implemented carefully.
Solutions:
- WebSockets (Socket.IO)
- Firebase Realtime DB
- MQTT for IoT-style communication
Pitfall: Keeping WebSocket connections alive with hundreds of thousands of users can kill your server — use load balancers and autoscaling groups.
7. Use Efficient State Management
Bad state management leads to re-renders, lags, and bugs in large apps.
Solutions:
- Jetpack Compose: Use
remember
,StateFlow
- SwiftUI: Use
@State
,@ObservedObject
- React Native: Redux or Context API
Tip: Keep UI, Logic, and Network layers strictly separate for better testing and scalability.
8. Monitoring, Logging & Analytics at Scale
You can’t fix what you can’t measure.
Must-Have Tools:
- Crashlytics or Sentry for real-time crash reporting
- Firebase Analytics, Mixpanel, or Amplitude for usage tracking
- Datadog or New Relic for performance monitoring
Case Study: Duolingo uses Mixpanel to understand user drop-off points and optimize flows.
9. Plan for Internationalization & Localization
As your app scales geographically, you’ll need to support multiple languages, currencies, and formats.
Best Practices:
- Use
NSLocalizedString
(iOS) orstrings.xml
(Android) - Date & number formats using
Intl
libraries - Dynamic layout support (RTL, font scaling)

10. Don’t Forget Security and Compliance
Scaling without security is asking for trouble.
Essentials:
- Use HTTPS always
- Store tokens securely (Keychain, Keystore)
- GDPR, HIPAA, or country-specific compliance
- Rate-limiting + 2FA for sensitive actions
Example: A fintech app like Revolut handles encrypted traffic, device binding, and biometric authentication for every session
Conclusion
Scalable mobile apps aren’t created by chance. They’re engineered with foresight — from database to UI layer. Whether you’re building for 1,000 or 10 million users, the principles of scalability must be baked in early.
By focusing on clean architecture, offline-first capabilities, modular components, analytics, and security, your mobile app will not just survive — it will thrive as it scales.